“Decision making” is one of the most important concepts of computer programming. Programs should be able to make logical (true/false) decisions based on the condition they are in; every program has one or few problem/s to solve; depending on the nature of the problems, important decisions have to be made in order to solve those particular problems.
In C programming “selection construct” or “conditional statement” is used for decision making. Diagram 1 illustrates “selection construct”.
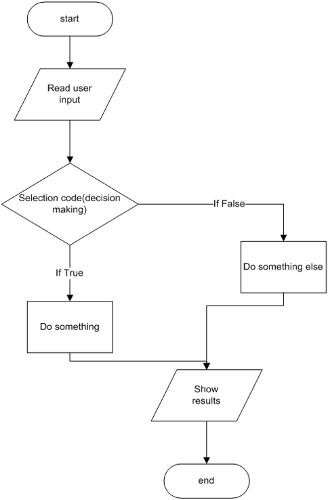
Diagram 1 simple selection construct
Conditional statement is the term used by many programming languages. The importance of conditional statements should not be ignored because every program has an example of these statements. “IF statement” and “switch statement” are the most popular conditional statements used in C.
Branching
Branch is the term given to the code executed in sequence as a result of change in the program’s flow; the program’s flow can be changed by conditional statements in that program. Diagram 2 shows the link between selection (decision making) and branching (acting).
Diagram 2 "branch" depends on the condition of the selection code
Branching is the process of choosing the right branch for execution, depending on the result of “conditional statement”.
If Statement
“If statement” is the selection statement used to select course of action depending on the conditions given. Therefore programmers can use this statement to control the flow of their program. If the program’s condition matches “if statement” condition then the code will be executed otherwise it will be ignored and the rest of the program will be executed.
Definition:
if (condition) { code here if “true”}
Tips :
{ } can be removed if only one line of code is to be executed. For example:
If(a==1)
Printf(“1”);
Instead of:
If(a==1)
{ printf(“1”); }
Example:
consider the case when we are asked to write a program for exforsys.com where users are asked to give ratings on each article; we want to output different messages depending on the ratings given. Messages are as follow: 1 is bad, 2 is average and 3 is good.
Code :
int rate;
printf("Please Enter Rating? 1 for bad , 2 for average and 3 for goodn");
scanf("%d",&rate);
if(rate==1)
{
printf("nit is poor");
}
if(rate==2)
{
printf("nit is average");
}
if(rate==3)
{
printf("nit is good");
}

Figure 1 code 1 screen shot
Description of “code 1”: this code asks user to input in first 3 lines, once the user inputs an integer the program will then checks all the “if statements”. If any of them match the input “rate” then the output for that statement will be printed out. Diagram 3 shows the function of the code 1.

{mospagebreak}
The If – Else Construct
“if else construct” is the upgraded version of “if statement”. Unlike “if statement” where you could only specify code for when condition is true; for “if else statement” you can also specify code for when the condition is not True (false). So the definition for the “if else statement” is:
Definition:
if(condition){ code when true } else { code when not true }
Tips :
{ } can be removed if only one line of code is to be executed. For example:
If(a==1)
Printf(“1”);
Else
Printf(“none”);
Instead of:
If(a==1)
{ printf(“1”); }
else
{ Printf(“none”);}
Example:
imagine the case where we need an input “greater than or equal to 5” and we want to warn the user if they use invalid input (less than 5). We can use the code below:
Code:
int number;
printf("Please Enter a number greater than or equal to 5?n");
scanf("%d",&number);
if(number>=5)
{
printf("nit is valid");
}
else
{
printf("nit is invalid");
}

Figure 2 code 2 screenshots
Compound Relational Tests
In order to check the conditions within the programs, C has number of relational operators. The table 1 summarises these operators using variables a=10 and b=5.
== |
Is Equal to – |
!= |
Not equal to |
> |
Greater than |
< |
Less than |
>= |
Greater than or equal to |
<= |
Less than or equal to |
Table 1 relational operators
Tips:
Relational operators can only compare two variables, for example: ‘a==a’ is correct, ‘a==a==b’ is incorrect syntax.
‘Logical operators’ are used in conditional statements such as ‘if statement’ to create a single testing condition. However some conditional statements require more than one testing conditions. For example: if we are asked to write program which output the word “yes” if the user’s input value is between 4 and 8 including number 8, we obviously can’t program this condition using only one testing condition; therefore we require logical operators to link those conditions. There are three logical operators: ‘!’,‘&&’ and ‘||’.
‘&&’ denotes to an operation of logical AND gate. ‘||’ denotes to an operation of logical OR gate. Table 2 and 3 describe the behaviour of && and || respectively.
Condition A |
Condition B |
Result |
0 |
0 |
0 |
0 |
1 |
0 |
1 |
0 |
0 |
1 |
1 |
1 |
Table 2 && operation
Condition A |
Condition B |
Result |
0 |
0 |
0 |
0 |
1 |
1 |
1 |
0 |
1 |
1 |
1 |
1 |
Table 3 || operation
Example #1: using && operator
Here we will refer back to the example we had before. We want to write a program which outputs “yes” if the user’s input is between 4 and 8 (including number 8). In order to write this we have to program the following condition in our ‘if statement’.
Input is greater than 4 AND less than or equal to 8.
Condition A Condition B
As you can see above, we have two conditions for our ‘if statement’; so we need to separate these two conditions in order to program the conditions in C.
Condition A is ‘greater than 4’; we program this in C assuming that the variable ‘A’ is the user input, now we have:
Condition A: A>4
We do the same for the second condition and we get:
Condition B: A<=8
Our two conditions are ready. We need to link them together using logical operators. For this example we need to use && operator. Therefore our final condition becomes (A>4 && A<=8).
Code:
int A;
printf("Please Enter a number greater than 4 and less than or equal to 8?n");
scanf("%d",&A);
if(A>4 && A<=8)
{
printf("nyes");
}

Figure 3 code 3
Description:
We have evaluated the written description of the problem and we produced the C code from that. As you can see above, the testing condition “A>4 && A<=8” is the same as mathematical expression “4
Hints:
Be extra careful with the way you format your conditions together. Using brackets to separate each condition from another is the best practice to avoid confusions for complex testing conditions. For example: we can write “A>4 && A<=8” as “(A>4) && (A<=8)”.
{mospagebreak}
Nested if Statement
Using “if…else statement” within another “if…else statement” is called ‘nested if statement’. “Nested if statements” is mainly used to test multiple conditions. They can be structured using following syntax:
If(conditionA)
{
Statements
}
Else if (conditionB)
{
statementB
}
Else
{
statement
}
Example #1:
Consider the case that you are asked to program a university marking system which identifies student’s grade on response to user input. Here is how grades should be grouped: 40+ E, 50-59 D , 60-69 C , 70-79 B , 80+ A
Code:
int Mark;
printf("Please Enter the grade between 0-100?n");
scanf("%d",&Mark);
if(Mark >= 40 && Mark <50)
{
printf("your grade is E n");
}
else if(Mark>=50 && Mark<60)
{
printf("Your grade is D n");
}
else if(Mark>=60 && Mark<70)
{
printf("Your grade is C n");
}
else if(Mark>=70 && Mark<80)
{
printf("Your grade is B n");
}
else if(Mark>=80 && Mark<90)
{
printf("Your grade is A n");
}else
printf("you have failed");

Figure 4 code 4 screenshot
Description:
This code checks from lowest boundary and checks for every grade, once one of conditions matches the mark the remaining conditions will be ignored. If none of the conditions match, it will then print out the message “you have failed”.The Switch Statement
“Switch statement” is another type of “conditional statement” used by programmers. Switch is widely used for menus.
Switch (variable)
{
Case 1: statement1 break;
Case 2: statement 2 break;
Case 3:statement 3 break;
.
.
.
Default: default statement break;
}
Tips:
“break” statement is very important. If it is not used properly all the code after the matched condition will be executed incorrectly. For example: looking at syntax 4; if the break for case 2 was missing, all the codes after and including case 2 would be executed until the next break statement was reached.
Example #1:
This example will cover a very basic character based menu that responds to a , b, c and d; it also warns the user if any other characters are used.
Code:
char response;
printf("Please Enter a,b,c or d?n");
scanf("%c",&response);
1:switch(response)
{
2:case 'a': printf("you have inputed 'a'"); break;
3:case 'b': printf("you have inputed 'b'"); break;
4:case 'c': printf("you have inputed 'c'"); break;
5:case 'd': printf("you have inputed 'd'"); break;
6:default: printf("unrecognised character"); break;
}

Figure: 5 simple menu
Description:
“statement 1” indicates that “switch” will be applied on ‘response’. “Statement 2” defines the action needed in case ‘response’ is holding the value of ‘a’. “statement 3,4,5” define action in case ‘response’ is holding value of ‘b’, ’c’ or ‘d’ respectively. “Statement 6” is defining the action needed if none of the cases meet the conditions.
About this tutorial
All codes presented in this tutorial are snippets therefore they will not work unless they are put into acceptable structure. Acceptable structure includes stdio.h library( or any other relevant library) and main() function in the program. All the snippets in this tutorial are tested by Microsoft Visual C++ Express 2010.
[catlist id=170]
.